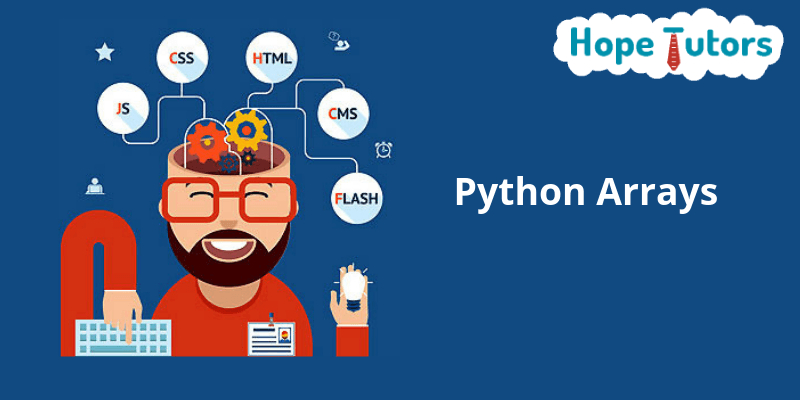
Python-Arrays: What is an Array and how we can use them?
Arrays are said to be one of the basic data structures. In simple words arrays are containers which hold fix number of items. All the items in the array should be in same data type. Lot of the DS will use arrays. This will help you in implementing their algorithms. Arrays help to reduce the burdens of the memory. Arrays are very fastest accessible elements.
Concept of arrays:
Following are the important terms in concept of Array. They are,
- Element
- Index
Element: An element is nothing other than each item stored in an array.
Index: Each location of an element in an array has a numerical index. It is used to identify the element.
Representing Array:
We can declare array in various ways in different languages. Arrays in Python will save us most of our time. As we already mentioned, arrays also help us in reducing the size. Python plays a major role in getting out of the problematic syntax.
Below is the best example for array.
As per the above example, following are some of the important points.
- Index starts with 0.
- Array length is 10 which means it can store 10 elements.
- Each element can access via its index.
Python Examples:
A=arr.array(‘d’,[1.4,1.5,2.3])
Array items help us for easy looping. We can fetch the necessary value. This is possible with the help of specification of the index number. The Arrays are very changeable. We can perform number of calculations as required.
The ‘array’ DS in the python core is not reliable. In python, array means lists.
Python provides Numpy Arrays. Data Science uses this Numpy arrays.
Basic Operations of an Array:
Following are the basic operations of an array. They are,
- Traverse: It will print all the array elements one by one.
- Insertion: It will add an element at the given index.
- Deletion: It will delete an element at the given index.
- Search: It will search an element using the given index.
- Update: It will update an element at the given index.
We can create an array in Python. This is possible by importing array module to the python program. Then we can declare the array.
Array Creation:
We can create an array in Python. This is possible once we import the array module.
-
- We can import the array as arr.
- This array functions have two parameters. They are as follows,
Data type.
Value list.
First, we should store the value of the data type. Then we should store the value lists. Data types may be anything like float, int, double and more. We mention arr as alias-name. It is just for easy use. We can also import without the alias. There is other way for importing this array module. It will be as follows,
- from the array import *
We can import all the functions wants from this array module.
Syntax for creating an array:
a=arr.array(data type,value list)
in other form
a=array(data type,value list)Examples:
b=arr.array( ‘d’ , [3.1 , 4.1 ,5.1] )
The first parameter: ‘d’. It will give the type of the data i.e. float. The specified values are the next parameters.
Python has various data types. The below table show the data types as well as the codes.
Type Codes Data Types Byte Size H Int 2 h Int 2 U Unicode character 2 i int 2 I int 2 d float 8 L int 4 l int 4 f float 4 Example for creating an Array:
from array import *
array1 = array(‘i’, [100,200,300,400,500])
for x in array1:
print(x)Compiling and executing the program the result will be as follows.
OUTPUT:
100
200
300
400
500Accessing the array element:
Index values should mention for accessing the array elements. Index number start at 0. They will not start from 1.
The index is less than 1 of the array lengths. We can access each element of an array using the index of the element.
Below is the syntax:
Array_Name [index value] Examples:
from array import *
array1 = array(‘i’, [100,200,300,400,500])
print (array1[0])
print (array1[2])Compiling and executing the program the result will be as follows. The result will show the element inserted at the index position 1.
OUTPUTS:
f100
300For Finding the Arrays Length:
The total number of the elements which presents in an array is the array length. We can use the len() function for achieving this. This function returns the integer value. This value equals the total number of the elements which present in the array.
Below is the syntax:
len(Array_name) Examples:
A=arr.array(‘d’, [1.10 , 2.10 ,3.10] )
len(A)OUTPUTS:
3 Its returns the value as 3 which equals to the total number of the array element.
Adding / changing an element of an arrays:
It is possible by adding the values to arrays. This is possible with the help of extend(), append() as well as insert (i,x) function.
append() is the function which use adding one element. Especially this is possible at the array end.
Examples:
A=arr.array(‘d’, [1.2, 2.2,3.2])
a.append(4.2)
print(A)OUTPUTS:
array(‘d’, [1.2, 2.2, 3.2, 4.2]) For adding more than one elements we must use extend() functions. This extend function takes the list of the element as parameters. This content of the list are elements which we add to this array.
Examples:
A=arr.array(‘d’, [1.10, 2.10 ,3.10])
a.extend([4.50,6.30,6.80])
print(A)OUTPUTs:
array(‘d’, [1.10, 2.10, 3.10, 4.50, 6.30, 6.80]) Insert operations is to insert one / more data into an array. This is possible with insert(i,x) functions. This function will insert elements at the corresponding index in the arrays. It will take 2 parameters. First parameter is index where the elements we need to insert. The second parameter is the values.
Examples:
from array import *
array1 = array(‘i’, [100,200,300,400,500])
array1.insert(1,600)
for x in array1:
print(x)OUTPUTS:
100
600
200
300
400
500Array Concatenation:
We can merge arrays by using the array concatenation. We can concatenate two arrays with the help of + symbol.
Examples:
A=arr.array(‘d’,[1.10,2.10,3.10,2.60,7.80])
B=arr.array(‘d’,[3.70,8.60])
C=arr.array(‘d’)
C=A+B
print(“Array C = “,C)OUTPUTS:
Array C= array(‘d’, [1.10, 2.10, 3.10, 2.60, 7.80, 3.70, 8.60]) Removing or to Delete the elements in an array:
We can remove array element by using remove() / pop() method. The major difference between the two functions is as follows,
-
- pop() will return the value which we delete.
- remove() will not return the deleted value.
The pop() will not take parameter or index values as its parameters. We can give no values to the parameter. At this case this function pop the last element & returns the value. When we give the value of an index, this function pop the needed elements. This function will return this.
Example:
A=arr.array(‘d’, [1.10, 2.20, 3.80, 3.10, 3.70, 1.20,4.60])
print(A.pop())
print(A.pop(3))OUTPUTS:
4.60
3.10The pop function removes last value 4.60. It will return the same also the second will pop the value in the 4th positions. The value is 3.10 & return the same.
The remove function uses to remove unneeded value. This will return the value after removing the unneeded value.
This function takes element values itself as the parameters. If you give the index value in the parameter, it will give an error.
Deletion refers to removing an existing element from the array. It will reorganize all the elements of an array.
Examples:
from array import *
array1 = array(‘i’, [100,200,300,400,500])
array1.remove(400)
for x in array1:
print(x)OUTPUTS:
100
200
300
500Slicing of an array:
We can slice the array with the help of : symbol. This returns the range of the elements that we specified by the index number.
Examples:
A=arr.array(‘d’,[1.10 , 2.10 ,3.10,2.60,7.80])
print(A[0:30])OUTPUTS:
array(‘d’, [1.10, 2.10, 3.10]) Looping the arrays:
We can loop the arrays. This is possible with the help of for loop.
Examples:
A=arr.array(‘d’, [1.10, 2.20, 3.80, 3.10, 3.70, 1.20, 4.60])
print(“All values”)
for x in A:
print(x)
print(“specific values”)
for x in A[1:3]:
print(x) -
OUTPUT:
All value 1.10 2.20 3.80 3.10 3.70 1.20 4.60 specific value 2.20 3.80 |
Search Operation:
We can search an array element based on its value or its index. This is possible with the help of index() function.
Example:
from array import * array1 = array(‘i’, [100,200,300,400,500]) print (array1.index(400)) |
OUTPUT:
300 |
Update Operation:
It means updating an existing element from the array. This is specially for the given index.
We reassign a new value to the desired index we want to update.
Example:
from array import * array1 = array(‘i’, [100,200,300,400,500]) array1[2] = 800 for x in array1: print(x) |
Compiling and executing the program the result will be as follows.
OUTPUT:
10 20 80 40 50 |
Here we have discussed all the concepts of array in Python. This come to the end of this article on Arrays in the Python. Keep in mind that practice makes a man perfect. So, keep on practicing. Keep updating your experience.