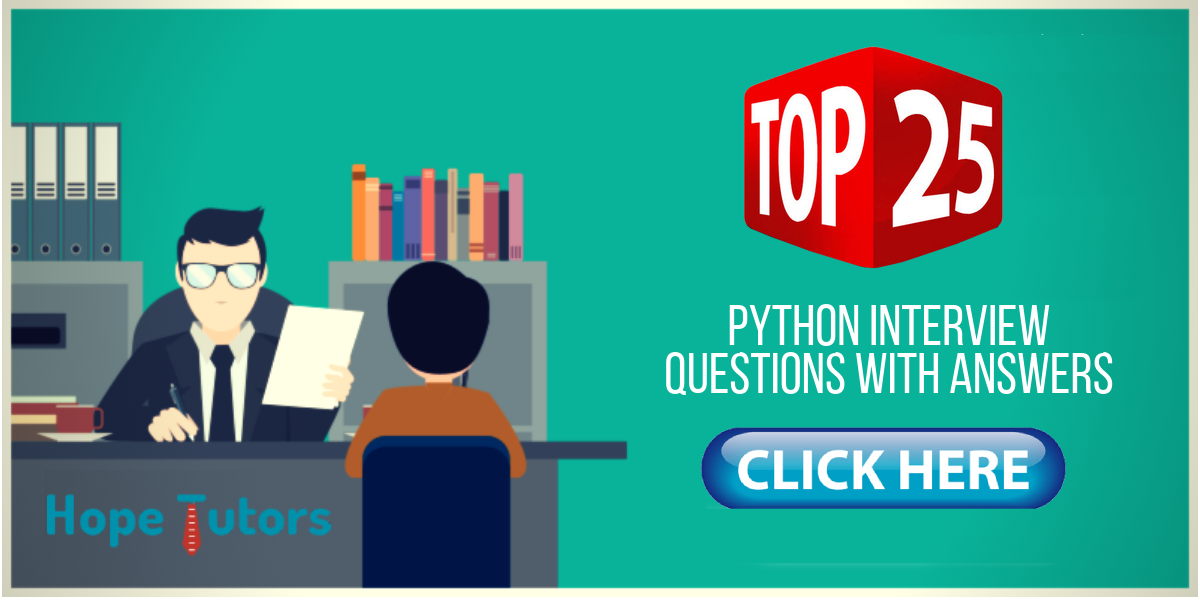
1. What do you think about Python and list its advantages?
Python is a best interpreted language. While writing python script, we don’t need compilation before executing the programs.
Advantages of Python:
- Python will support object-oriented programming. We can able to define classes with the inheritance and composition..
- In python language, functions stand as first-class object. Functions could be assigned to variables.
- It is a dynamic language. We don’t need to mention the data type of the variables during declaration.
- Developing the code with the help of Python is very easy and quick. Running the code is comparatively slow than compilation of language often.
- It gives flexibility to include the C programming language extension. It will help us in optimizing the script.
- Python languages has vast number of usages such as web-based apps, big data analytics, test-automation and data-modeling.
2. List some of the Built-in-types in Python?
Following are some of the most commonly used python supported built-in-type. They are,
Immutable built-in-datatype of Python
- Numbers
- Strings
- Tuples
Mutable built-in-datatype of Python
- List
- Dictionaries
- Sets
3. How will you find the Bugs in a Python Applications?
PyChecker is static analysis tool. We can use this to identify bugs in the python applications. It will also reveal the complexity and style related issues. The other tool used is Pylint. It is useful to check whether Python modules fulfills coding-standard.
4. What is mean by PEP-8?
PEP-8 is the advanced standard in Python. It is a coding convention. Simply said as a coding recommendation which will guide us in delivering more readable code.
5. When will you use the Python Decorator?
Python Decorator refers to changes which we can apply to the Python syntax in order to adjust the functions faster.
6. What is mean by Pickling as well as unpickling?
Pickling is a process. The process will involve the following steps. They are,
- Pickling modules will accept any python object.
- It will convert it into the string representation.
- Then dumps it into a file by using the dump functions.
Unpickling is also a process. It is used to retrieve the original Python object from the stored string representation.
7. How will you state the principle different between The Lambda and Def?
S.NO | Lambda | Def |
1 | It has an uni-expression functions. | It can hold a multiple expression |
2 | It can form a functional object and return the value to it. | It will generate a function and destinates a name which can be called later. |
3 | It does not have any return statement. | It will have return statement. |
8. How memory management is done in Python?
- Python will use a private heap it will helps in maintaining its memory. The Python data structures and objects are stored in heap. Python interpreter could access it. The Programmers can use it.
- The Memory-manager in Python will handle private-heap. It allocates the required memory for the python object.
- Python involves in garbage collector (inbuilt) which would salvage unused memory as well as free the space to heap.
9. State the principle difference between the List and the Tuple?
The principle difference between the List and the Tuple is that the former is mutable in the List whereas it is not mutable in the Tuple.
The Tuple can be hashed. For example: It is used as a key for the dictionaries.
10. Can you state the difference between the deep as well as shallow copy?
Shallow copy: It is used mainly when we create the new instance type and keep the values which we copy them in new instance. This is used for coping the refence pointers. It is just like coping the values. These references the object-oriented point. These are the changes made with any of the members in class will affect the copy of the original value. It will allow the faster program execution. It will depend the size of the used data.
Deep Copy: It is used the value which is already copied. It will not copy the refence pointer to the object. Instead it will make references to the object. New object which pointed by another object will get stored. These changes in the original copies will not affect the other copies which uses the objects. It will make the program execution slower. This is because of making the certain copies each object which is called.
11. How will you achieve multi-threading in Python?
- Python has package of multi-threading. It has the construct called Global Interpreted Lock (GIL). This will make sure that one of the threads can be executed at any single time. The thread with the GIL has little work than passes GIL to next thread.
- It will happen quickly so that for us it may seemed to be threads can be executed parallelly, but it is just taking turn using same core of the CPU.
- All GIL will pass the add overhead to the execution. If we want to run the code faster, then this usage of multi-threading is not good idea.
12. What is pass in?
Pass: It means Python statement with no-operation. It can be simply said as Place holder in compound statement where there is a blank and nothing is written there.
13. How can you use ternary operators in Python?
This operator is used for showing the conditional statement. This will contain true / false value with the statement which is to evaluate for this.
Syntax:
Syntax of ternary operation is
[on_true] if [exp] else [on_false]a,b=10,20big=a if a |
Example:
This expression gets evaluated like x<y else y, in this case if x<y is true then the value is returned as big=x and if the value is false then big=y will be generated as the result.
14. What is inheritance in Python?
It will allow one class for gaining all members of the other class. It will provide the code reusability which will make easy to create & maintain an app. The inherited class is known as super class. Similarly, a class we derive from the super class is derived or child class.
There is different type of inheritance. They are as follows,
- Single inheritance – The derived class which has the member of the single super class.
- Multi-level inheritance – The derived class d1 is inherited from base class b1 and d2 is inherited from b2.
- Hierarchical inheritance – We can inherit any number of child class from a single base class.
- Multiple inheritance – We can inherit a derived class from more than one base class.
15. What is string and docstring in Python?
String: A String is a sequence of alpha numeric characters. They are generally immutable objects. They will not allow to modify the value after assignment. There are several methods like join(), split(), replace() or to change the string. But it will not change the original value.
Docstring: Documentation string is known as docstring. Simply, it is a way to document the python functions, modules and classes.
16. What is mean by Slicing and Generators in Python?
Slicing: Slicing is a powerful method used to select a range of items from list, tuple, strings etc.
Generators: Generators is the way of implementing the iterators. It is a usual function except it yields expression in the functions.
17. Explain the built-in function which python uses to iterate over a number sequence?
Range() produces numbers as a list. To iterate the loop, these numbers are useful.
for i in range(50):
print(j)
|
The function range() facilitates two set of function parameters.
- range(stopvalue)
- stopvalue:It specifies the number of values (integers) to produce. It starts from 0. For example, range(5) meant to range [0,1,2,3,4].
- range([startvalue], stop[,stepvalue])
- startvalue:Initial number to start the sequence.
- Stop:It specifies the higher (upper) limit of sequence.
- Stepvalue:Increment factor to generate sequence.
Only the arguments of type integer are allowed. Parameters could be negative or positive. The range() function will start from 0th index in Python programming.
17. What is meant by the dictionary in the Python state with an example?
The dictionary is the built-in datatype in the python. It will define the 1-1 relationship between the keys & values. It will contain the pair of the keys & their corresponding value. It is indexed by the keys.
Examples:
The following is the example which contain some keys. Mother, Father and Child. The values are Manju, Ganesh and Kuttima respectively.
1 | dict={ ‘Mother’: ‘Manju’, ‘Father’: ‘Ganesh’ Child’ : ‘Kuttima’}
1 | print dict[mother] Manju 1 | print dict[Father] Ganesh 1 | print dict[child] Kuttima
|
18. What is mean by unittest in Python?
It is a unit test framework in Python. It will support the sharing of setups, automation setting, shut down code for test, aggregation of tests into collections etc.
19. What do you mean by negative index in Python?
In Python, sequences can be index either in positive as well as in negative numbers. For the positive index the first index will be 0, the second index will be 1 and goes on. For the negative index the last index will be -1, the second index will be -2 and goes on.
20. Explain % S in Python?
Python will support the formatting any value into string. It has contained the quite complex expression. Pushing values into string is done with the help of %S format specifier is one of the most common usage. The formatting operation has the comparable syntax as in C function has printf().
21. What is mean by monkey patching in Python explain with an example?
It means dynamic modification of the class / a module during run time.
Example:
#monkeyPatching.py class MonkeyPatchingClass: def func(self): print “func()”
|
Monkey Patching testing would be
import monkeyPatching def monkey_func(self): print “monkey_func()” monkeyPatching.MonkeyPatchingClass.func = monkey_func obj = monkeyPatching.MonkeyPatchingClass() obj.func()
|
The result will be as follows,
monkey_func()
There are changes introduced to the behavior of func() in MonkeyPatchingClass with the function we defined, monkey_func(), outside of the module monkeyPatching.
22. Write a 1-line code to count the no of small letters in the file. This code must work even when the files are big?
A solution written in multiple lines could be replaced as single lining solution
with open(VERYLARGEFILE) as largefile: counter = 0 textStore = largefile.read() for fileCharacter in textStore: if fileCharacter.islower(): counter += 1
|
The above multi line solution could be changed as below
count sum(1 for line in largefile for fileCharacter in line if fileCharacter.islower())
23. How can you perform copying object in Python?
copy.copy() or copy.deepcopy() is used for copying object in the Python. It is used for general case. It does not support to copy all objects, but we can able to copy most of them.
24. State the differences between Xrange and range?
Range: It will return the list. It will use the same memory irrespective of the range size.
Xrange: It will return the xrange object.
25. What will you say about module and package in the Python?
Module:It is the simple way to structure the programs. Each Python file will have module which will import the other modules like objects and attributes.
Package: It is the collection of folders or modules. The folder of python programs will contain the package of the modules.
27. What are all different ways to clear the list in python?
#1. Using clear() method
# Python program to clear a list
# using clear() method # list of letters GEEK = [6, 0, 4, 1] print(‘GEEK before clear:’, GEEK) # clearing vowels GEEK.clear() print(‘GEEK after clear:’, GEEK)
|
#2. Reinitializing the list
# Python3 code to demonstrate
# clearing a list using # clear and Reinitializing # Initializing lists list1 = [1, 2, 3] list2 = [5, 6, 7] # Printing list1 before deleting print (“List1 before deleting is : ” + str(list1)) # deleting list using clear() list1.clear() # Printing list1 after clearing print (“List1 after clearing using clear() : ” + str(list1)) # Printing list2 before deleting print (“List2 before deleting is : ” + str(list2)) # deleting list using reinitialization list2 = [] # Printing list2 after reinitialization print (“List2 after clearing using reinitialization : ” + str(list2))
|
#3. Using “*= 0”
# Python3 code to demonstrate
# clearing a list using # *= 0 method # Initializing lists list1 = [1, 2, 3] # Printing list1 before deleting print (“List1 before deleting is : ” + str(list1)) # deleting list using *= 0 list1 *= 0 # Printing list1 after *= 0 print (“List1 after clearing using *= 0: ” + str(list1))
|
#4. Using del
# Python3 code to demonstrate
# clearing a list using # del method # Initializing lists list1 = [1, 2, 3] list2 = [5, 6, 7] # Printing list1 before deleting print (“List1 before deleting is : ” + str(list1)) # deleting list1 using del del list1[:] print (“List1 after clearing using del : ” + str(list1)) # Printing list2 before deleting print (“List2 before deleting is : ” + str(list2)) # deleting list using del del list2[:] print (“List2 after clearing using del : ” + str(list2))
|