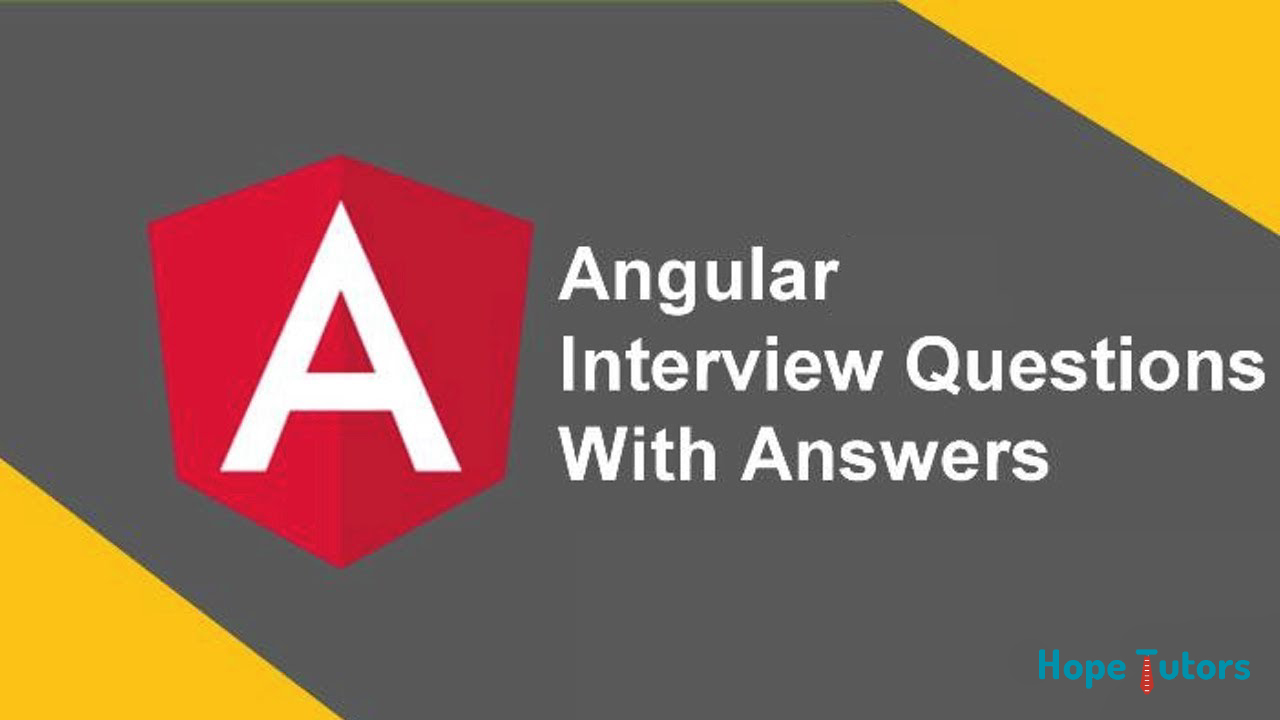
Angular Interview Questions and Answers
1. What is new in the Angular ?
Angular Elements will be enabled to support content projection. This is done with the help of web standards for custom elements.
Angular Material Gets Minor Updates
Angular Material will get better display. It will give in an elegant look in the new update. Moreover, it will also add a new homepage for the material, material.io. In this, we will get tooling, design guidance, development components and stay up-to-date with the latest news.
If we are using an Angular Material then we can observe a visual difference as library can make changes to itself. This is done with the updated version of the Material design
Better Accessibility for Selects
In the updated version, it will include a lot of new features to enhance accessibility for selects. It will add a new feature of the native select inside mat-form-field. It is so far better and outperformed than the mat- select.
Both the select and mat-select are available so we can choose what we want to do.
Virtual Scrolling
The Component Dev Kit (CDK) is available in the market with the great virtual scrolling capabilities that the user can apply by importing the `ScrollingModule`!
<cdk-virtual-scroll-viewport itemSize="20">
<div *cdkVirtualFor="let dog of dogsArray"> {{dog}}</div>
</cdk-virtual-scroll-viewport>
- Drag & Drop
The CDK in the new update also now recommend to Drag & Drop. This will possess these great hallmarks.
-
Automated render as a user moves items
It is a new feature available only in Angular 7.
-
Helper methods for reordering/transferring items in lists
For reordering or transferring items in lists, we can use a helper method. They are moveItemInArray and transferArrayItem.
-
Enhancing Application Performance
We will get enhanced application performance in Angular 7.
-
A safeguard has come into play for the users of Angular 7
It gives a portent to new application builders when they are crossing the budget with their bundle size. The warning occurs on 2 MB whereas an error occurs over 5 MB. But we don’t need to frighten. We can change the limits simply in our angular.json file. The thing we must do is add in a bit about the warnings and error sizes with budget details.
“budgets”: [
{
“type”: “initial”,
“maximumWarning”: “2mb”,
“maximumError”: “5mb”
}
]
2. What do you mean by Angular Framework?
In simple words we can say that angular is a TypeScript-based open-source front-end platform. It is very easy to build applications with in web, mobile and desktop. The major features of this framework are declarative templates, dependency injection, end to end tooling etc., which are used to ease the development.
3. What do you mean by TypeScript?
TypeScript is a typed superset of JavaScript. It is created by Microsoft. It will add optional types, classes, async or await, and many other features. It will compile to plain JavaScript. Angular built entirely in TypeScript and used as a primary language.
4. How can you install TypeScript globally?
We can install TypeScript globally as follows
npm install -g typescript
Let us see a simple example of TypeScript usage,
function greeter(city: string)
{
return “Welcome, ” + city;
}
let user = “Chennai+”;
document.body.innerHTML = greeter(user);
5. How will you update angular versions to latest?
Following are the simple steps to update the angular version. They are,
- First, we have to update the Angular version globally. This is possible by inserting the latest version via the terminal: sudo npm install -g @angular/cli@latest.
- The next step is to upgrade the version locally in our project. We need to assure the altering for the new version are displayed in the package.json file ng update @angular/cli.
- When it iscompleted, upgrade all our dependencies and dev dependencies in package.json.
- To build Angular applications, Angular-devkit was introduced in Angular 6. It will need the dependency on the CLI projects.
- With all of this, we will require upgrading the version for Typescriptnpm install [email protected] –save-dev.
- Then, we need to relocate the configuration of angular-cli.json to angular.json ng update @angular/cli and ng update @angular/core.
- Use this command: ng update @angular/material in case of Angular material is used.
- The next step is the removal of deprecated RxJS 6 features npm install -g rxjs-tslint rxjs-5-to-6-migrate -p src/tsconfig.app.json.
- When it is completed, uninstall rxjs-compat as it is not required for Angular npm uninstall –save rxjs-compat.
- Also change import {Observable} from ‘rxjs/Observable’; to import {Observable} from ‘rxjs’;
- Finally, we can start our Angular 7 application by using ng serve.
6. Can you state the difference between AngularJS and Angular?
S:NO | AngularJS | Angular |
1 | This will be based on MVC architecture. | This will be based on Service/Controller. |
2 | This will use JavaScript to build the application. | It Introduced the typescript to write the application. |
3 | It is Based on controller concept. | It is a component-based UI approach. |
4 | It is not a mobile friendly framework. | It is developed considering mobile platform. |
5 | Difficulty in SEO friendly application development. | Ease to create SEO friendly applications. |
7. Can you give an example of a simple HTML document with some header information and page content?
HTML documents are different. They will follow a basic structure of head and body. Here we are providing a simple document structure and basic tags such as DOCTYPE, html, head, title, meta, body, h1, p, etc.
For example:
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
<meta charset=”UTF-8″>
<meta name=”description” content=”Page description”>
</head>
<body>
<h1>Hope Tutors</h1>
<p>Angular 7 Course</p>
</body>
</html>
8. What is your understanding about the CSS box model? Write some code to describe?
In simple words we can say that CSS is a language which will describe how the webpages look. The CSS box model will refer to the layout and design of HTML elements. It is in box shape which will wrap around each HTML element. A box is made up of the following elements. They are,
- Content of the box
- Padding
- Border
- Margin
Condition 1: The same padding on all 4 sides.
padding: 25px;
Condition 2: padding for the top, right, bottom, left.
padding: 25px 50px 75px 100px;
Condition 3: Top/bottom padding 25 pixels, right/left padding 50 pixels.
padding: 25px 50px;
9. How will you change the style of an HTML element using JavaScript?
Let us see with an example to change the font size.
document.getElementById(“someElement”).style.fontSize = “18”;
10. Can you write some of the code for a basic class in TypeScript with a constructor and a method?
Here we are going to see the simple class that is created with a greeting message which can be retrieved with the greet () function.
class Greeter
{
greeting: string;
constructor(message: string)
{
this.greeting = message;
}
greet()
{
return “Hello, ” + this.greeting;
}
}
let greeter = new Greeter(“world”);
11. What do you mean by Single Page Applications? How do they work in Angular?
In short term Single Page Applications is SPAs. These are web applications. It will use only one HTML page. As the user interacts with the page, new content is dynamically updated on that master page. Navigation between pages happens without refreshing the whole page. Angular uses AJAX and to dynamically update HTML elements. Angular Routing can be used to make SPAs. The result is an application that feels more like a desktop app rather than a webpage.
12. Can you draw the pictorial diagram of Angular architecture?
The following diagram shows the architectural model of angular
13. Can you list the key components of Angular?
Following are the key components of angular. They are,
- Component: These are the basic building blocks of angular application. It is used to control HTML views.
- Modules: An angular module is set of angular basic building blocks. They are like component, directives, services etc. An application is divided as logical pieces. Each piece of code is called as “module”. It will perform a single task.
- Templates: This will represent the views of an Angular application.
- Services: It is used to create components. This will be shared across the entire application.
- Metadata: This will be used to add more data to an Angular class.
14. What do you mean by directives?
Directives will add the behaviour to an existing DOM element or an existing component instance.
import { Directive, ElementRef, Input } from ‘@angular/core’;
@Directive({ selector: ‘[myHighlight]’ })
export class HighlightDirective {
constructor(el: ElementRef) {
el.nativeElement.style.backgroundColor = ‘yellow’;
}
}
Now this directive extends HTML element behavior with a yellow background as below
<p myHighlight>Highlight me!</p>
15. What do you mean by components?
Components are the most basic UI building block of an Angular app. It will form a tree of Angular components. These components are subset of directives. Unlike these directives, components always have a template and only one component can be instantiated per an element in a template. Let’s see a simple example of Angular component
import { Component } from ‘@angular/core’;
@Component ({
selector: ‘my-app’,
template: ` <div>
<h1>{{title}}</h1>
<div>Learn Angular6 with examples</div>
</div> `,
})
export class AppComponent {
title: string = ‘Welcome to Angular world’;
}
16. List out the differences between Component and Directive?
S:NO | Components | Directives |
1 | To register a component, we should use @Component meta-data annotation. | To register directives, we should use @Directive meta-data annotation. |
2 | Components are used to create UI widgets. | Directives are used to add behavior to an existing DOM element. |
3 | Components are used to break up the application into smaller components | Directives are used to design re-usable components. |
4 | Only one component can be present per DOM element . | Many directives can be used per DOM element. |
5 | @View decorator or templateurl/template are mandatory. | Directive doesn’t use View. |
17. What do you mean by template?
In simple term we can say that a template is a HTML view. Using this we can able to display the data by binding controls to properties of an Angular component. You can store your component’s template in one of two places. You can define it inline using the template property, or you can define the template in a separate HTML file and link to it in the component metadata using the @Component decorator’s templateUrl property. Using inline template with template syntax,
import { Component } from ‘@angular/core’;
@Component ({
selector: ‘my-app’,
template: ‘
<div>
<h1>{{title}}</h1>
<div>Learn Angular</div>
</div>
‘
})
export class AppComponent {
title: string = ‘Hello World’;
}
Using separate template file such as app.component.html
import { Component } from ‘@angular/core’;
@Component ({
selector: ‘my-app’,
templateUrl: ‘app/app.component.html’
})
export class AppComponent {
title: string = ‘Hello World’;
}
18. What do you mean by a module?
Modules are logical boundaries in your application. The application will be divided into separate modules to separate the functionality of your application. Let us see with an example of app.module.ts root module declared with @NgModule decorator as below,
import { NgModule } from ‘@angular/core’;
import { BrowserModule } from ‘@angular/platform-browser’;
import { AppComponent } from ‘./app.component’;
@NgModule ({
imports: [ BrowserModule ],
declarations: [ AppComponent ],
bootstrap: [ AppComponent ]
})
export class AppModule { }
The NgModule decorator has three options. They are,
- The imports option is used to import other dependent modules. The Browser Module is required by default for any web based angular application.
- The declarations option is used to define components in the respective module.
- The bootstrap option tells Angular which Component to bootstrap in the application.
19. How will you handle the events in the angular 7?
There are various methods to handle events in Angular 7. These are:
- Binding to user input events: You can use the Angular event binding to answer to DOM event. User input will trigger so many DOM events. It is a very effective method to get the input from the user.
For example,
<button (click)=”onClickMe()”>Click me!</button>
- Get user input from the event object: DOM will carry a cargo of the information that possibly is valuable for the components. Here is an example to show you the keyup event of an input box to obtain the user’s input after every stroke.
src/app/keyup.components.ts (template v.1)
content_copy
template: `
<input (keyup)=”onKey($event)”>
<p>{{values}} </p>
- 3Key event filtering: Every keystroke is heard by the (keyup) event handler. The enter keys matter the most as it provides the sign of the user that he has done with the typing. The most efficient method of eliminating the noise is to look after every $event. keyCode and the action is taken only when the enter key is pressed.
20. What are Core Dependencies of angular 7?
There are two type of core dependencies. They are RxJS and TypeScript.
- RxJS 6.3:
RxJS version 6.3 is used by Angular 7. It has no changes in the version from Angular 6.
- TypeScript 3.1
TypeScript version 3.1 is used by Angular 7. It is the upgrade from the version2.9 of Angular 6.
21. Explain about Bazel?
Bazel is one of the best test tools. It is just like the Make, Maven and Gradle. It is an open-source build. Bazel utilizes readable and high-level build language. It will handle the project in a great number of languages and builds the product for many platforms. Moreover, it will support multiple users and large codebases over several repositories.
22. How to create a decorator in Angular?
There are two ways to register decorators in Angular. These are as follows.
- $provide.decorator.
- module.decorator.
23. What do you mean by IVR Render? Is it supported by angular 7?
Angular will be releasing a new kind of rendering pipeline and view engine.
The purpose of angular view engine is to translate the templates as well as components that we have written into the regular HTML and JavaScript. It is very easy for the browser to read it comfortably. Ivy is believed to be splendid for the Angular Renderer.
Yes, it is supported by Angular 7.
24. How will you generate the class in angular 7 using CLI?
To create a class we should use the below code:
ng generate class <name> [options]
ng g class <name> [options]
Where name refers the name of a class.
Options refer to the project name, spec value in Boolean or type of a file.
25. Can you state the difference between Structural and attribute directives in angular?
Structural directives are used to alter the DOM layout. This is done by removing and adding DOM elements. It is far better in changing the structure of the view. Best examples of Structural directives are NgFor and Nglf.
Attribute Directives These are being used as characteristics of elements. For example, a directive such as built-in NgStyle in the template Syntax guide is an attribute directive.
26. What are the available lifecycle hooks?
Angular application goes through an entire set of processes or has a lifecycle right from its initiation to the end of the application. The representation of lifecycle in pictorial representation as follows.
The description of each lifecycle method is as below,
- ngOnChanges: When the value of a data bound property changes, then this method is called.
- ngOnInit: This is called whenever the initialization of the directive/component after Angular first displays the data-bound properties happens.
- ngDoCheck: This is for the detection and to act on changes that Angular can’t or won’t detect on its own.
- ngAfterContentInit: This is called in response after Angular projects external content into the component’s view.
- ngAfterContentChecked: This is called in response after Angular checks the content projected into the component.
- ngAfterViewInit: This is called in response after Angular initializes the component’s views and child views.
- ngAfterViewChecked: This is called in response after Angular checks the component’s views and child views.
- ngOnDestroy: This is the cleanup phase just before Angular destroys the directive/component.
27. What do you mean by data binding?
Data binding is a core concept in Angular. It will allow to define communication between a component and the DOM. It is very easy to define interactive applications without worrying about pushing and pulling data. There are four forms of data binding. This will be divided as 3 categories which differ in the way the data is flowing.
- From the Component to the DOM: Interpolation: {{ value }}: Adds the value of a property from the component
<li>Name: {{ user.name }}</li>
<li>Address: {{ user.address }}</li>
Property binding: [property]=”value”: The value is passed from the component to the specified property or simple HTML attribute
<input type=”email” [value]=”user.email”>
- From the DOM to the Component: Event binding: (event)=”function”: When a specific DOM event happens (eg.: click, change, keyup), call the specified method in the component
<button (click)=”logout()”></button>
- Two-way binding: Two-way data binding: [(ngModel)]=”value”: Two-way data binding allows to have the data flow both ways. For example, in the below code snippet, both the email DOM input and component email property are in sync
<input type=”email” [(ngModel)]=”user.email”>
28. What do you mean by metadata?
Metadata is used to decorate a class. It can configure the expected behavior of the class. The metadata is represented by decorators.
- Class decorators, e.g. @Component and @NgModule
import { NgModule, Component } from ‘@angular/core’;
@Component({
selector: ‘my-component’,
template: ‘<div>Class decorator</div>’,
})
export class MyComponent {
constructor() {
console.log(‘Hey I am a component!’);
}
}
@NgModule({
imports: [],
declarations: [],
})
export class MyModule {
constructor() {
console.log(‘Hey I am a module!’);
}
}
- Property decorators Used for properties inside classes, e.g. @Input and @Output
import { Component, Input } from ‘@angular/core’;
@Component({
selector: ‘my-component’,
template: ‘<div>Property decorator</div>’
})
export class MyComponent {
@Input()
title: string;
}
- Method decorators Used for methods inside classes, e.g. @HostListener
import { Component, HostListener } from ‘@angular/core’;
@Component({
selector: ‘my-component’,
template: ‘<div>Method decorator</div>’
})
export class MyComponent {
@HostListener(‘click’, [‘$event’])
onHostClick(event: Event) {
// clicked, `event` available
}
}
- Parameter decorators Used for parameters inside class constructors, e.g. @Inject
import { Component, Inject } from ‘@angular/core’;
import { MyService } from ‘./my-service’;
@Component({
selector: ‘my-component’,
template: ‘<div>Parameter decorator</div>’
})
export class MyComponent {
constructor(@Inject(MyService) myService) {
console.log(myService); // MyService
}
}
29. What do you mean by angular CLI?
In full form we can say that angular CLI is Command Line Interface. It is a command line interface to scaffold and build angular apps using nodejs style (commonJs) modules. We need to install using below npm command,
npm install @angular/cli@latest
Following are the few commands which will come handy while creating angular projects.
Creating New Project: ng new
Generating Components, Directives & Services: ng generate/g the different types of commands are,
- ng generates class my-new-class: add a class to your application.
- ng generates component my-new-component: add a component to your application.
- ng generates directive my-new-directive: add a directive to your application.
- ng generates enum my-new-enum: add an enum to your application.
- ng generates module my-new-module: add a module to your application.
- ng generates pipe my-new-pipe: add a pipe to your application.
- ng generates service my-new-service: add a service to your application.
- Running the Project: ng serve.
30. State the difference between constructor and ngOnInit?
TypeScript classes has a default method called constructor. It is normally used for the initialization purpose. Whereas ngOnInit method is specific to Angular, especially used to define Angular bindings. Even though constructor getting called first, it is preferred to move all our Angular bindings to ngOnInit method. In order to use ngOnInit, we need to implement OnInit interface as below,
export class App implements OnInit{
constructor(){
//called first time before the ngOnInit()
}
ngOnInit(){
//called after the constructor and called after the first ngOnChanges()
}
}
31. What do you mean by service?
A service is used when a common functionality needs to be provided to various modules. Services will allow for greater separation of concerns for our application and better modularity by allowing you to extract common functionality out of components. Let us create a repoService which can be used across components,
import { Injectable } from ‘@angular/core’;
import { Http } from ‘@angular/http’;
@Injectable({ // The Injectable decorator is required for dependency injection to work
// providedIn option registers the service with a specific NgModule
providedIn: ‘root’, // This declares the service with the root app (AppModule)
})
export class RepoService{
constructor(private http: Http){
}
fetchAll(){
return this.http.get(‘https://api.github.com/repositories’);
}
}
32. What is mean by dependency injection in Angular?
Dependency injection in short form DI. It is an important application design pattern in which a class asks for dependencies from external sources rather than creating them itself. Angular comes with its own dependency injection framework. It is used for resolving dependencies. So, we can have our services depend on other services throughout our application.
33. What is the basic syntax of a Decorator in Angular?
ANS: @() with optional parameters.
34. For what [(ngModel)] will be used for?
In simple words we can tell this as two-way data binding.
35. Can you tell me the basic parts of an Angular application?
Following are some of the basic parts of the Angular. They are,
- Modules.
- Component.
- Data Binding.
- Template.
- Directives.
- Dependency Injection.
- Services.
- Routing.
36. Tell me the purpose of async pipe?
The AsyncPipe is about subscribing to an observable or promise and returns the latest value it has emitted. Whenever a new value is emitted then the pipe marks the component to be checked for changes. It will take a time to observable which continuously updates the view for every 2 seconds with the current time.
@Component({
selector: ‘async-observable-pipe’,
template: `<div><code>observable|async</code>:
Time: {{ time | async }}</div>`
})
export class AsyncObservablePipeComponent {
time = new Observable(observer =>
setInterval(() => observer.next(new Date().toString()), 2000)
);
}
37. Can you tell me the option to choose between inline and external template file?
We can store your component’s template in one of two places. We can define it inline using the template property. we can also define the template in a separate HTML file and link to it in the component metadata. This is done by using the @Component decorator’s templateUrl property.
The choice between inline and separate HTML is a matter of taste, circumstances, and organization policy. But normally we use inline template for small portion of code and external template file for bigger views. By default, the Angular CLI generates components with a template file. But you can override that with the below command,
ng generate component hero -it
38. Can you tell me the purpose of ngFor directive?
We use Angular ngFor directive in the template. This is to display each item in the list. For example, here we iterate over list of users,
<li *ngFor=”let user of users”>
{{ user }}
</li>
The user variable in the ngFor double-quoted instruction is a template input variable.
39. Can you tell me the purpose of ngIf directive?
In sometimes an app needs to display a view or a portion of a view only under specific circumstances. The Angular ngIf directive inserts or removes an element. It will be based on a true or false condition. Best example to display a message if the user age is more than 18,
<p *ngIf=”user.age > 18″>You are not eligible for student pass!</p>
40. What will happen if you use script tag inside template?
Angular recognizes the value as unsafe and automatically sanitizes. It will remove the <script> tag but keeps safe content such as the text content of the <script> tag. This way it eliminates the risk of script injection attacks. If you still use it then it will be ignored. A warning appears in the browser console. Best example of innerHtml property binding which causes XSS vulnerability,
export class InnerHtmlBindingComponent {
// For example, a user/attacker-controlled value from a URL.
htmlSnippet = ‘Template <script>alert(“0wned”)</script> <b>Syntax</b>’;
}
41. What do you mean by interpolation?
Interpolation is a special syntax. Using this Angular will convert into property binding. It’s a convenient alternative to property binding. It is represented by double curly braces ({{}}). The text between the braces is often the name of a component property. Angular replaces that name with the string value of the corresponding component property. The best example,
<h3>
{{title}}
<img src=”{{url}}” style=”height:30px”>
</h3>
In the example above, Angular evaluates the title and url properties and fills in the blanks, first displaying a bold application title and then a URL.
42. List some advantages of using Angular framework for building web applications?
Following are some of the advantages of using angular framework for building web applications. They are,
- It will save lot of time for developers. This is possible by doing a lot of the work for them like writing tedious DOM manipulation tasks.
- TypeScript and the Angular framework will allow us to catch errors much earlier.
- In many cases has faster performance than traditional web development techniques.
- Can give web apps the feel of a desktop application.
- It separates out the code of an application to make it easier for multiple developers to work on an app and easier to test.
- More consistent code base which will be easy to maintain.
- Big developer community.
43. What function will be called when an object is created in TypeScript? What is it basic syntax in TypeScript code?
The constructor function will be called.
It‘s syntax is: Constructor(){}
44. How will you interact between Parent and Child components in angular?
When passing data from Parent to Child component, we can use the @Input decorator in the Child component. Similarly, when passing data from Child to Parent component, we can use the @Output decorator in the Child component.
45. Can you give me an example usage of ngFor for displaying all items from an array ’Items‘ in a list with <li>?
Following will be the best example.
<li *ngFor=”let item of Items”>
{{item}}
</li>
46. List the main difference between constructor and ngOnInit?
The constructor is a feature of the class. It is not an Angular. The main difference is that Angular will launch ngOnInit. Once it is finished configuring the component, then it is a signal through which the @Input() and other banding properties and decorated properties are available in ngOnInit, but are not defined within the constructor by design.
47. What are HTTP Interceptors?
Interceptor is just a fancy word for a function that receives requests or responses before they are processed or sent to the server. You should use interceptors if you want to pre-process many types of requests in one way. For example, you need to set the authorization header Bearer for all requests
token.interceptor.ts
import { Injectable } from ‘@angular/core’;
import { HttpInterceptor, HttpRequest, HttpHandler, HttpEvent } from ‘@angular/common/http’;
import { Observable } from ‘rxjs/Observable’;
@Injectable()
export class TokenInterceptor implements HttpInterceptor {
public intercept(req: HttpRequest<any>, next: HttpHandler): Observable<HttpEvent<any>> {
const token = localStorage.getItem(‘token’) as string;
if (token) {
req = req.clone({
setHeaders: {
‘Authorization’: `Bearer ${token}`
}
});
}
return next.handle(req);
}
}
And register the interceptor as singleton in the module providers:
app.module.ts
import { NgModule } from ‘@angular/core’;
import { BrowserModule } from ‘@angular/platform-browser’;
import { HTTP_INTERCEPTORS } from ‘@angular/common/http’;
import { AppComponent } from ‘./app.component’;
import { TokenInterceptor } from ‘./token.interceptor’;
@NgModule({
imports: [
BrowserModule
],
declarations: [
AppComponent
],
bootstrap: [AppComponent],
providers: [{
provide: HTTP_INTERCEPTORS,
useClass: TokenInterceptor,
multi: true // < – – – – an array of interceptors can be registered
}]
})
export class AppModule {}
48. How many Change Detectors can there be in the whole application?
Each component has its own ChangeDetector. All Change Detectors are inherited from AbstractChangeDetector.
49. List out some of the important practices to secure an Angular application?
Following are some of the important practices to secure an angular application. They are,
- Check that all requests come from within your own web app and not external websites.
- Sanitize all input data.
- Use Angular template instead of DOM APIs.
- Content Security Policies.
- Validate all data with server-side code.
- Use an offline template compiler.
- Avoid including external URLs in your application.
- Make JSON responses non-executable.
- Keep all libraries and frameworks up-to-date.
50. What do you mean by ViewEncapsulation and how many ways are there do to do it in Angular?
In simple words, ViewEncapsulation determines whether the styles defined in a component will affect the entire application or not. Angular supports 3 types of ViewEncapsulation. They are
- Emulated – Styles used in another HTML spread to the component.
- Native – Styles used in another HTML doesn’t spread to the component.
- None – Styles defined in a component are visible to all components of the application.